API-driven boarding quickstart
This guide covers setting up a custom app for processing boarding applications using example code, including modifying the form layout, applying a custom theme, and changing individual form fields. The result is a custom app that’s catered towards private corporations with a fulfillment window of 30 days or less on all transactions and a minimum average transaction amount of $100 USD.
Hosting your own boarding solution means that you’re responsible for maintaining it. Talk to your Payabli solutions engineer about whether API-driven boarding is a good option for your organization.
Visit the repository’s README for information about specific files, functions, component props, and other technical details.
Dependencies
Before you begin, make sure you have the following installed on your machine:
Set up the app
Run these commands in your terminal to configure the boarding example app on your local machine:
Fill in your environment variables
Open the .env
file in your code editor. Set PAYABLI_API_TOKEN
to your Payabli API token and BETTER_AUTH_SECRET
to any text string with a length of 32 characters:
The BETTER_AUTH_SECRET
is a string used to encrypt sensitive information.
You can generate a 32-character string using the secret key generator in the better-auth documentation.
The BETTER_AUTH_URL
is the base URL of your app.
Authentication
The example boarding app uses a JavaScript library called better-auth for user authentication. Authentication is important because it prevents access to secure information by unauthorized users.
Move form fields
The <WizardStep>
component represents one step in the form wizard, and its code contents are displayed as the form’s current tab.
By default, the form has several steps:
- Business Information
- Business Details
- Contacts & Ownership
- Financial Information
- Payment Information
The bsummary
(business summary) field is in the “Financial Information” step. Let’s move it to the “Business Information” step.
Find the "Financial Information" section
In your code editor, navigate to src/components/PayabliForm.tsx
. Find the <WizardStep>
component with a label
value of "Financial Information"
.
Delete the bsummary
form field
Under the <WizardStep>
component, delete the <FormInput/>
component with a name
value of "bsummary"
:
Find the "Business Information" section
Scroll up and find the <WizardStep>
component with a label
of "Business Information"
:
Change section details
Let’s give the “Business Information” section a new name and a new icon.
Find the "Business Information" section
Go back to the <WizardStep>
component with a label
of "Business Information"
:
Change step props
Change the label
prop to "Corporation Overview"
, then change the icon
prop from {<Building />}
to {<Briefcase />}
:
Edit header title to match
Finally, change the <h2>
element below to match the new label
prop: “Step 1: Corporation Overview”:
Save changes
Your <WizardStep>
component should say “Corporation Overview” and have a briefcase icon.
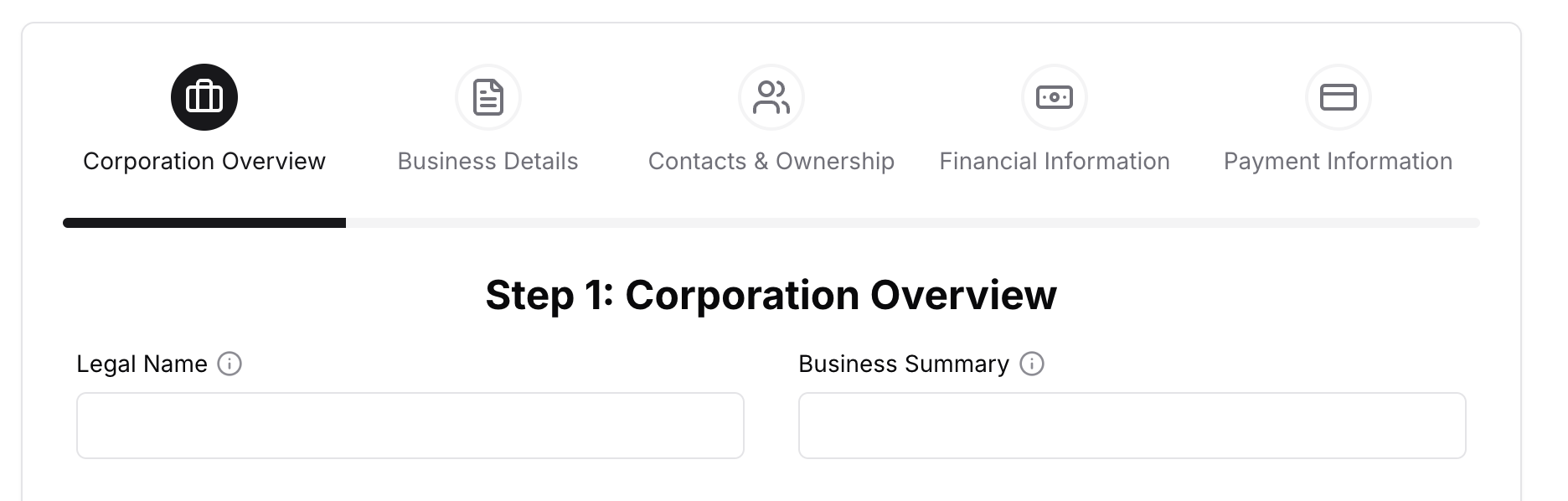
The icons in this example app use a library called
lucide. You can find a list of all available icons on their website.
Lucide icons must be imported at the top of the file. Scroll to the top of the file and find
the import list from lucide-react
. Add a new line at the top of the list that says Briefcase,
.
Add form validation rules
Form fields need to validate input data. For example, the email
field should have a valid email.
If the user enters an invalid email, the form should display an error message.
The form schema in src/Schema.ts
contains the validation rules.
The Schema.ts
file is also where the templateId
field is located. If you need to change the boarding template, change the value inside of the .default()
method.
We need to add a validation rule to prevent the form from accepting an average transaction value less than $100 USD.
Entering a value less than 100 in the ticketamt
field now displays an error message.
Remove form fields
Let’s remove the form field that asks for the business type, because your application is only used by private corporations.
Remove the form field component
In src/components/PayabliForm.tsx
, delete the <FormSelect/>
component with the name
prop set to "btype"
:
All new submissions will set the btype
field to a value of "Private Corp"
.
Removing form fields and providing default values is effectively the same as pre-filling the form field with a default value, as can be done with Payabli’s hosted boarding solutions. See Prefill Applications.
Customize form inputs
Because the ticketamt
form field doesn’t accept values less than 100, we need to tell the user that there is a minimum amount of $100 USD.
We can do this by editing the <FormInput/>
component for the ticketamt
field.
To configure autocompletion for form fields, such as for addresses, see the README. We recommend using an address autocompletion service like Google Places or Smarty for production applications.
Customize form selects
Because this app is for private corporations with a fulfillment window of 30 days or less, we need to edit the whenDelivered
and whenRefunded
form fields.
To learn more about the available props for the form components, see the README.
Customize shadcn/ui themes
The example boarding application is built with a UI library called shadcn/ui. This makes the application easy to customize with themes.
Find the theme styles
In your code editor, navigate to src/styles/globals.css
. The first @layer base { ... }
block determines the theme.
Open the shadcn/ui theme generator
Instead of manually editing all of the values, you can use the shadcn/ui theme builder to generate them for you.